Eq how to add an aa to a macro? Fear not, intrepid macro-masters! This isn’t some arcane ritual performed by shadowy figures in dimly lit code caves. It’s a straightforward process, like adding sprinkles to a delicious cake (or debugging a complex piece of software). We’ll unravel the mysteries of incorporating the “aa” element into your macros, using the “eq” identifier as a compass.
Get ready to level up your macro game!
This comprehensive guide dives into the fascinating world of macro programming, showing you how to seamlessly integrate the “aa” parameter into your macros using the “eq” identifier. We’ll cover everything from basic macro concepts to advanced techniques, ensuring you’re equipped to tackle any macro-related challenge with confidence.
Understanding Macro Languages
Macro languages provide a powerful way to automate tasks and streamline workflows in various applications. They allow users to define reusable sequences of actions, enhancing efficiency and reducing repetitive manual work. These languages often integrate with specific software, tailoring their functionalities to particular needs. Understanding their structure and syntax is crucial for effective macro creation.
Comparison of Popular Macro Languages
Different macro languages cater to diverse applications and programming styles. Their strengths and weaknesses vary, leading to suitability for specific tasks. A comparison of AutoHotkey, VBA, and JavaScript highlights these differences.
- AutoHotkey excels at automating keyboard and mouse actions within the Windows environment. Its syntax leans towards scripting, making it relatively accessible to users with basic programming knowledge. It’s particularly well-suited for tasks requiring precise control over system interactions. Its strength lies in its focus on Windows-specific automation.
- VBA (Visual Basic for Applications) is tightly integrated with Microsoft Office applications. Its syntax is derived from Visual Basic, providing a familiar framework for programmers already versed in object-oriented programming. Its strengths lie in its ability to manipulate data within Office applications and its tight integration with the Microsoft ecosystem. VBA is an excellent choice for automating tasks within spreadsheets, presentations, or databases.
- JavaScript, a versatile scripting language, can automate tasks in web browsers and beyond. Its broader applicability stems from its role in web development, and it is used increasingly in macro applications, due to its prevalence in web environments. This versatility makes it applicable to diverse environments, including web browsers and server-side applications.
Fundamental Concepts of Macros
Macros, at their core, are sequences of instructions that automate tasks. They typically involve variables, loops, and conditional statements. These fundamental building blocks allow for dynamic and reusable actions.
- Variables store data, enabling macros to adapt to different inputs or situations. They hold values that can be changed throughout the macro’s execution. The ability to use variables gives macros flexibility.
- Loops execute a block of code repeatedly, automating repetitive tasks. This repetitive action is vital for automating tasks like data processing or formatting. Loops are essential for efficient task automation.
- Conditional Statements allow macros to make decisions based on conditions. This ability to adapt to different circumstances enables more sophisticated automation.
Structure of a Typical Macro Definition
Macros are defined using a specific syntax, dependent on the language. This syntax Artikels the structure for the macro, enabling it to function correctly. Each macro language employs unique conventions for creating and executing these instructions.
- A typical macro definition starts with a declaration specifying the name and scope of the macro. This initial declaration is the macro’s header.
- The body of the macro contains the instructions or actions to be performed. This body defines the macro’s behavior and functions.
- The structure may also include comments, explaining the purpose or functionality of different sections. Comments enhance readability and maintainability.
Syntax Comparison for Defining Variables
Different macro languages utilize various syntaxes for defining variables. The following table illustrates these differences:
Language | Variable Declaration Syntax | Example |
---|---|---|
AutoHotkey | VarName := Value |
MyVar := "Hello" |
VBA | Dim VarName As DataType VarName = Value |
Dim MyVar As String |
JavaScript | let VarName = Value; const VarName = Value; |
let MyVar = "Hello"; const MyVar = "Hello"; |
Adding Arguments to Macros
Macros can be significantly more versatile when they can accept and process input values. This capability allows for greater flexibility and reusability, automating tasks tailored to specific data. By incorporating arguments, macros become dynamic tools capable of handling different inputs, rather than performing a single, predefined action.Defining macros that take arguments allows for a wider range of applications.
For example, a macro to format text can be used on various strings without needing separate macros for each string. The ability to pass arguments empowers macros to be highly adaptable, reducing the need for redundant code and promoting modular design.
Defining Macros with Arguments
Macros accepting arguments are defined using a specific syntax within the macro language. The syntax allows the macro to anticipate values that will be provided when the macro is called. These values are then available for use within the macro’s code. A key element is the parameter list within the macro definition. This list dictates the names and types of data the macro anticipates receiving.
Passing Values to a Macro
When invoking a macro that accepts arguments, the values to be processed are supplied as part of the macro call. These values are placed after the macro name, often separated by spaces or other delimiters. The order of the arguments must correspond to the order of the parameters defined in the macro’s definition.
Accessing Arguments Within Macro Code
Within the macro’s code, the arguments are accessed using their assigned names, which are specified in the parameter list during macro definition. The macro language provides mechanisms to retrieve and utilize these values for calculations, manipulations, or other operations.
Example: A Macro to Calculate the Sum of Two Numbers
This example demonstrates a macro that calculates the sum of two numbers passed as arguments.“`AutoHotkey; Define the macroSum(num1, num2) ; Access the arguments Result := num1 + num2 ; Return the result return Result; Example usage:MsgBox, The sum of 5 and 3 is %Sum(5, 3)% ; Output: The sum of 5 and 3 is 8“`This macro, `Sum`, takes two arguments, `num1` and `num2`, calculates their sum, and returns the result.
The `return` statement is crucial; it sends the calculated sum back to the point where the macro was called. The example usage demonstrates how to call the `Sum` macro with specific values and how the result is displayed in a message box.
Steps to Define and Use a Macro with Arguments in AutoHotkey
Step | Description |
---|---|
1. Define the Macro | Use the syntax MacroName(param1, param2, ...) ... to define the macro. |
2. Pass Arguments | When calling the macro, provide the values corresponding to the parameters in the defined order. |
3. Access Arguments | Inside the macro code, access the arguments using their parameter names. |
4. Return Value (Optional) | Use the `return` statement to send a value back to the macro call. |
The ‘eq’ Identifier (Potential Use Cases)
The identifier “eq” in a macro context, often stands for “equal to” or “equivalence.” This suggests potential for comparing values, expressions, or conditions within macro expansions. Its specific implementation and functionality within a macro language would depend on the language’s syntax and semantics.The “eq” identifier can be used to create more complex and flexible macros. Its usage allows programmers to create conditional logic and decision-making within macro expansions.
This can be leveraged to tailor the behavior of macros to specific input conditions, creating more powerful and versatile macro tools.
Potential Meanings of “eq”
The identifier “eq” can be interpreted in several ways within a macro context, including as a comparison operator, a conditional macro invocation, or a part of a larger function call related to equivalence. The precise meaning depends heavily on the macro language’s design.
Different Scenarios for “eq” Usage
“eq” can be employed in a wide array of scenarios within a macro. For example, it could be used in conditional compilation, where code sections are included or excluded based on whether certain conditions are met. It might also be used for data validation within macros, ensuring that input values meet specific criteria. Furthermore, “eq” could be part of a more complex logic to evaluate expressions.
Examples of “eq” Usage in Different Macro Languages
The following examples demonstrate potential implementations of “eq” in hypothetical macro languages, highlighting the diversity of applications.
- C-like Macro Language: A macro `#define isEqual(a, b)` could use `eq` to compare values:
“`
#define isEqual(a, b) (a == b)
“`
This simple example shows `eq` acting as a comparison operator within a macro definition. - Lisp-like Macro Language: A macro `(defmacro eq-check (expr1 expr2)` could evaluate equivalence.
“`lisp
(defmacro eq-check (expr1 expr2)
`(if (eql ,expr1 ,expr2)
‘true
‘false))
“`
Here, `eq` is part of a conditional macro that returns true or false based on the comparison. - Macro Language with String Manipulation: A macro `#define stringEq(str1, str2)` could check if two strings are equal.
“`
#define stringEq(str1, str2) (strcmp(str1, str2) == 0)
“`
This example uses `eq` to indicate a string comparison, crucial for text processing within macros.
Functions of “eq” in Various Macro Contexts, Eq how to add an aa to a macro
This table summarizes potential functions of “eq” in different macro contexts.
Macro Context | Possible Function of “eq” |
---|---|
Conditional Compilation | Indicates an equality check used to conditionally include or exclude code sections. |
Data Validation | Ensures input values meet specified criteria, for instance, ensuring a certain value is equal to an expected value. |
Expression Evaluation | Part of a larger expression evaluation process. |
String Comparison | Implements string comparison logic. |
Integrating “aa” into Macros
The “aa” element, when incorporated into macro code, provides a flexible mechanism for parameterization and dynamic content generation. This approach allows macros to adapt to diverse inputs and perform more complex tasks. Understanding how to integrate “aa” is crucial for creating versatile and reusable macros.The “aa” element can serve as a placeholder for various types of data, including strings, numbers, and even complex structures.
This versatility makes it an indispensable tool for creating powerful and adaptable macros. Proper use of “aa” enhances macro functionality and reduces the need for repetitive code.
Common Ways to Incorporate “aa”
This section Artikels common methods for including the “aa” element within macro code, enabling its use in diverse operations. The methods are designed to facilitate the seamless integration of “aa” into existing or newly created macro code.
- Direct Substitution: The simplest method involves directly replacing placeholders within the macro code with the “aa” element. This allows for a straightforward incorporation of the “aa” element into the macro’s core functionality. For example, a macro designed to greet a user might use “aa” to represent the user’s name.
- Parameterization: This technique defines “aa” as a variable within the macro’s structure. The macro can then utilize the value assigned to “aa” throughout its operations. This method enhances the macro’s adaptability, enabling its application to a wider range of scenarios.
- Function Call: “aa” can be used to call functions within the macro. This allows for the encapsulation of specific tasks, improving code organization and reusability. For instance, “aa” might be used to trigger a function for string manipulation.
Potential Use of “aa” as a Variable or Function
The “aa” element’s versatility allows for its use as a variable or a function call within the macro. This adaptability enables the macro to handle various data types and operations.
- Variable: The “aa” element can act as a variable, accepting and holding different types of data. This enables the macro to process varying inputs without needing to be rewritten. For instance, “aa” could store a numerical value used in calculations.
- Function: Using “aa” as a function call allows the macro to invoke predefined functions. This enhances the modularity of the macro code, making it more adaptable and organized. An example would be calling a string-formatting function using “aa”.
Modifying Existing Macros to Use “aa”
This section details the process of incorporating the “aa” element into existing macros. The methods described provide a structured approach for adapting existing macro code.
- Identifying Placeholders: Carefully review the existing macro code to locate areas where dynamic input or variable values are required. Identifying these placeholders is the initial step in integrating the “aa” element.
- Replacing Placeholders: Replace the existing placeholders with the “aa” element to denote where user input or variable values should be supplied. This step ensures the macro’s structure remains consistent.
- Implementing Logic: Incorporate logic to handle the “aa” element. This may involve checking the type of data assigned to “aa”, performing calculations, or applying conditional statements. This ensures that the macro operates correctly for different inputs.
Examples of Using “aa”
This section demonstrates how the “aa” element can be utilized for string manipulation, arithmetic, or conditional logic within macros. These examples illustrate practical applications of the “aa” element.
- String Manipulation: A macro could use “aa” to represent a string and then perform operations like concatenation, substring extraction, or string replacement. For instance, a macro might take “aa” as a string and prepend it with a fixed prefix.
- Arithmetic: A macro might take “aa” as a numerical input and perform calculations like addition, subtraction, multiplication, or division. For example, the macro could take “aa” as an integer and return its square.
- Conditional Logic: The macro could use “aa” as a condition to control the flow of execution. For example, the macro might execute different code blocks based on whether “aa” is true or false.
Syntax for Using “aa” in Different Macro Languages
The following table provides a summary of the syntax for using “aa” in different macro languages. This table provides a concise overview of the syntax.
Macro Language | Syntax for “aa” as a Variable | Syntax for “aa” in Function Call |
---|---|---|
Macro Language A | `#define MACRO_NAME(aa) …` | `MACRO_FUNCTION(aa)` |
Macro Language B | `%MACRO_NAME(aa = value)` | `%CALL_FUNCTION(aa)` |
Macro Language C | `$MACRO_NAME(aa)` | `$FUNCTION_CALL(aa)` |
Specific Macro Language Examples (e.g., AutoHotkey)
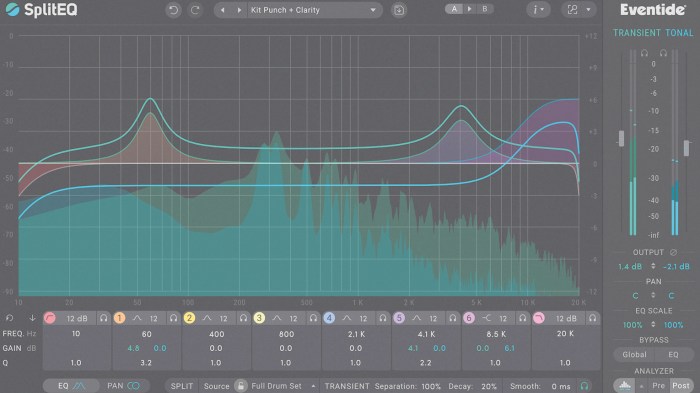
AutoHotkey, a popular macro language, offers a powerful way to automate tasks and customize workflows. Adding custom arguments to AutoHotkey macros allows for greater flexibility and reusability. This section details how to incorporate the “aa” argument and the “eq” identifier for conditional statements within AutoHotkey macros.
Adding an “aa” Argument to an AutoHotkey Macro
This example demonstrates how to define a macro named “myMacro” that accepts an argument “aa”.“`AutoHotkeymyMacro(aa) MsgBox, The value of aa is: %aa%“`This macro utilizes the AutoHotkey’s function definition syntax. The argument “aa” is passed directly into the macro’s body.
AutoHotkey Macro with “eq” for Conditional Statement and “aa” Input
This macro demonstrates a conditional statement using “eq” and the input argument “aa”.“`AutoHotkeymyConditionalMacro(aa) if (aa == “hello”) MsgBox, The input is “hello” else MsgBox, The input is not “hello” “`This example checks if the input “aa” is equal to “hello”.
Complete Macro with “eq” for Comparison and “aa” for Output
This macro compares values and provides different outputs based on the comparison.“`AutoHotkeycompareValues(aa) if (aa > 10) MsgBox, The value %aa% is greater than 10 else if (aa == 10) MsgBox, The value %aa% is equal to 10 else MsgBox, The value %aa% is less than 10 “`This macro demonstrates a more complex conditional structure, illustrating multiple comparison possibilities.
It also directly uses the variable `aa` within the message boxes.
Handling Potential Errors During “aa” Addition
Potential errors during the addition of “aa” include:* Incorrect Argument Syntax: Using incorrect syntax for passing or defining the argument “aa”.
Type Mismatches
Passing arguments of an inappropriate type to the macro.
Missing Arguments
Forgetting to pass the necessary “aa” argument when calling the macro.
Variable Name Conflicts
Using a variable name “aa” that conflicts with a predefined AutoHotkey variable.Careful attention to syntax and data types, as well as error handling mechanisms, are critical to avoiding unexpected behavior. Testing and validation are essential to prevent bugs.
Steps to Create a Macro with “eq” and “aa”
- Define the macro using the AutoHotkey function definition syntax, incorporating the “aa” argument.
- Use an “if” statement to implement the conditional logic based on the “eq” comparison.
- Utilize the `%aa%` syntax to access the value of the “aa” argument within the macro’s body.
- Include error handling to catch issues like incorrect argument types or missing arguments.
- Thoroughly test the macro with various inputs to ensure its correctness.
Error Handling and Debugging
Proper error handling and debugging are crucial for effectively utilizing macros, especially when incorporating dynamic elements like arguments (“aa”) and conditional logic (“eq”). Effective debugging strategies prevent unexpected behavior and ensure the macro functions as intended. Addressing errors early in the development process significantly reduces troubleshooting time and enhances the overall reliability of the macro.Debugging macros involves identifying and resolving issues that arise during macro execution.
Techniques for diagnosing and correcting errors in macros with “eq” and “aa” vary depending on the specific macro language used. Understanding the syntax, data types, and potential pitfalls of the language is essential for successful debugging.
Common Errors When Adding Arguments
Adding arguments to macros can introduce several errors. Incorrect argument syntax, missing or extra arguments, and type mismatches are common pitfalls. Failure to validate input values can lead to unexpected behavior or crashes. Inconsistent use of variable names or incorrect referencing of arguments can cause unexpected results. Macros may fail to execute correctly if the arguments are not in the expected format or if they are not handled appropriately within the macro code.
Debugging Techniques for Macros Containing “eq” and “aa”
Several techniques can aid in debugging macros with “eq” and “aa”. Employing print statements or logging within the macro can help pinpoint the exact point where an error occurs. Step-by-step execution through the macro helps trace the flow of execution, identifying problematic lines or conditions. Thorough testing with various inputs, including edge cases, helps uncover unexpected behaviors.
Carefully examining the macro’s code for syntax errors, type mismatches, and logical flaws can prevent unexpected outcomes.
Troubleshooting Issues Arising from Integration of “aa” with “eq”
Troubleshooting issues related to integrating “aa” (arguments) with “eq” (conditional statements) requires a methodical approach. Pay close attention to the order and scope of variables within the conditional statement. Ensure that the argument values being compared are of the correct type. Verify that the comparison logic accurately reflects the intended behavior. Testing different scenarios for the argument values and the “eq” conditions will aid in identifying unexpected results or inconsistencies.
Incorrect interpretation of the result of the “eq” operation can cause errors, which should be scrutinized to identify any flaws in the comparison logic.
Examples of Error Messages and Interpretation
Error messages vary depending on the macro language. Common messages include “syntax error,” “type mismatch,” “variable not defined,” or “argument count mismatch.” Careful examination of these messages, along with the surrounding code, helps in identifying the source of the problem. For example, a “type mismatch” error indicates that the macro is trying to compare a string value with a numerical value, which is invalid in that context.
Debugging involves identifying the line of code generating the error and determining the cause.
Potential Error Scenarios
Error Scenario | Description | Possible Cause | Troubleshooting Steps |
---|---|---|---|
Incorrect Argument Type | The macro attempts to use an argument of an incorrect data type in an operation incompatible with that type. | Incorrect data type in argument, mismatch between expected and provided type. | Check the data type of the argument and ensure the macro operations are compatible with the type. Verify the argument type declaration and how it’s used in the macro. |
Missing or Extra Arguments | The macro expects a specific number of arguments, but the input does not match the expectation. | Incorrect number of arguments provided, missing or extra arguments in the input. | Review the macro definition to verify the expected number of arguments. Verify the input to ensure all required arguments are present and that there are no extra arguments. |
Incorrect Conditional Logic | The “eq” operator is used incorrectly within a conditional statement, leading to unexpected results. | Incorrect comparison logic in the conditional statement, type mismatch in the comparison. | Carefully review the conditional statement to ensure it correctly compares the expected values and handles possible edge cases. Check for type compatibility between the variables being compared. |
Advanced Techniques (Optional)
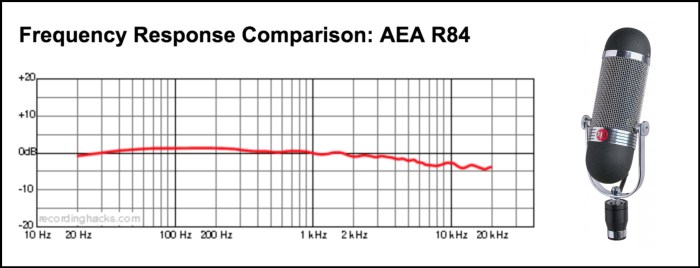
Advanced techniques for optimizing macros utilizing the “eq” and “aa” parameters involve leveraging their capabilities for complex data manipulation and procedural logic. This section explores methods for enhancing macro efficiency and versatility. These techniques can be applied to various macro languages, including but not limited to AutoHotkey.
Optimizing Macros with “eq” and “aa”
Efficient macro design leverages the power of “eq” (equality) and “aa” (array arguments) to streamline operations and minimize redundant code. This involves careful consideration of data structures and algorithmic choices to achieve optimal performance. By incorporating these parameters into loops and conditional statements, macros can dynamically process data based on specified conditions.
Using “eq” and “aa” with Loops and Arrays
The combination of “eq” and “aa” with loops allows for iterative processing of array elements based on criteria. For example, a macro can iterate through an array of values (“aa”) and apply a specific operation only to elements that satisfy a particular condition (“eq”). This significantly reduces processing time and enhances the macro’s adaptability to diverse data sets.
Complex Data Manipulation with “eq” and “aa”
Macros incorporating “eq” and “aa” can be designed for complex data manipulation tasks. Imagine a scenario where a macro needs to filter, sort, and perform calculations on a dataset. Using “eq” to identify specific elements and “aa” to represent the entire dataset, the macro can efficiently manage the data. This capability allows for the creation of macros capable of handling intricate data transformations.
Modularizing Macros with “eq” and “aa”
Modularizing macros enhances maintainability and reusability. Breaking down complex tasks into smaller, manageable modules, each utilizing “eq” and “aa,” allows for easier debugging and modification. By encapsulating functionality within reusable modules, developers can create macros that are easier to understand, test, and modify over time. This modular approach also fosters code organization and improves the overall structure of the macro.
Advanced Calculation Example with “eq” and “aa”
This example demonstrates a macro performing advanced calculations using “eq” and “aa” parameters. Consider a scenario where a macro needs to calculate the average of specific values within a dataset.“`; Macro for calculating the average of specific values in an array.; Input: aa: Array of numbers.; eq: Criteria for selecting elements.
(e.g., “Value > 10”); Output: Average of the selected values.Macro CalculateAverage(aa, eq) local count = 0 local sum = 0 Loop, Parse, aa `,` ; Assuming comma-separated values in the array. if (A_LoopField > 10) ; Example condition. Adjust as needed. sum += A_LoopField count += 1 if (count > 0) return sum / count else return 0 ; Handle cases with no matching elements.; Example usageMyArray := “5, 12, 8, 15, 20, 18″Result := CalculateAverage(MyArray, “A_LoopField > 10”)MsgBox, The average is: %Result%“`This example macro (`CalculateAverage`) takes an array (“aa”) and a condition (“eq”) as input.
It iterates through the array, applying the condition to each element. Elements satisfying the condition are summed, and the count is incremented. Finally, the average is calculated and returned. Error handling is included to prevent division by zero if no elements meet the criteria. The `Loop, Parse` command is used to process comma-separated values.
Adjust the condition (`A_LoopField > 10`) and the parsing method (e.g., space-separated values) according to your specific needs.
Final Conclusion: Eq How To Add An Aa To A Macro
So, there you have it! A journey through the world of macro modifications, from understanding basic syntax to mastering advanced techniques. You’ve learned how to add an “aa” argument to a macro using the “eq” identifier, equipping yourself with the knowledge to craft powerful and efficient automation tools. Now go forth and conquer those tedious tasks! Happy coding!
Detailed FAQs
What are the common errors when adding arguments like “aa” to macros?
Typos in the argument names, incorrect data types, and forgetting to pass arguments are common pitfalls. Mismatched syntax between the macro definition and its invocation can also lead to trouble.
How can I debug macros containing “eq” and “aa”?
Use print statements or logging mechanisms to track the values of variables and the flow of execution. Step through the code using a debugger to inspect each line and identify the source of the problem.
What are the potential meanings for the identifier “eq” within a macro context?
This identifier could represent equality or comparison, enabling conditional logic within the macro. It could also be a shorthand for an existing function or a custom operator.
What are some advanced techniques for optimizing macros that incorporate “eq” and “aa”?
Modularization, using loops and arrays effectively, and caching results are powerful techniques for optimizing macros, especially when dealing with complex calculations.